When building a web app, selecting the right database is one of the most important decisions you’ll make. It’s not just about storing data. It’s about speed, scalability, and reliability. For Sharepon, MySQL stood out as the best fit. Thanks to its robust performance, extensive community support, and versatility, MySQL has become a cornerstone of modern web development.
However, choosing MySQL is just the beginning. To truly unlock its potential, you need strategies to optimize its performance and ensure your queries run efficiently. In this post, I’ll share why I chose MySQL for Sharepon and the key optimization techniques that keep it running smoothly.
Relational vs. Non-Relational Databases
Databases generally fall into two categories: relational and non-relational. Relational databases, like MySQL, organize data into tables with rows and columns, using predefined schemas to maintain consistency. Relationships between data are established through primary and foreign keys, similar to linking rows across multiple spreadsheets.
Non-relational databases, such as MongoDB, are more like JSON objects, storing data in flexible, hierarchical structures. They’re ideal for unstructured or semi-structured data, allowing nested fields and varying schemas. This flexibility makes non-relational databases well-suited for real-time analytics, content management systems, or other use cases involving diverse data formats.
While non-relational databases excel in specific scenarios, relational databases remain the gold standard for structured data and complex relationships. Features like ACID compliance ensure consistency and reliability, making them a trusted choice for applications requiring transactional integrity, such as banking or inventory management.
For Sharepon, the data naturally fits a relational format. For example:
- A user table stores user attributes like usernames and emails, where each row is a user and each column is a user attribute.
- A coupon table records coupon codes and expiration dates, where each row is a coupon and each column is a coupon attribute.
- Relationships, such as users saving coupons to collections, can be represented in an intermediate table with columns for user_id, coupon_id, and collection_date.
This structure allows for efficient data storage and queries, making the overall data layout easy to follow and maintain. Even if Sharepon evolves to a point where a relational database alone isn’t sufficient, a non-relational database could be introduced to handle specific workloads. While maintaining two databases adds complexity, careful design and abstraction layers can unlock performance and scalability benefits.
Why I Chose MySQL
As of 2024, MySQL and PostgreSQL are the two most widely used relational databases in terms of market share. While both are powerful, MySQL’s simplicity, speed, and extensive community support made it the right choice for Sharepon. For new developers or those less familiar with database administration, MySQL’s vast community and abundant resources—tutorials, forums, and documentation—make it easier to learn and troubleshoot.
PostgreSQL, while feature-rich and capable of handling complex queries and custom data types, often has a steeper learning curve. Although it also has a strong community, MySQL’s focus on ease of use makes it a more approachable option for many developers.
Tip: When choosing a database, study its limits and FAQ pages in the manual to understand its performance boundaries and suitability for your project.
Database Optimization
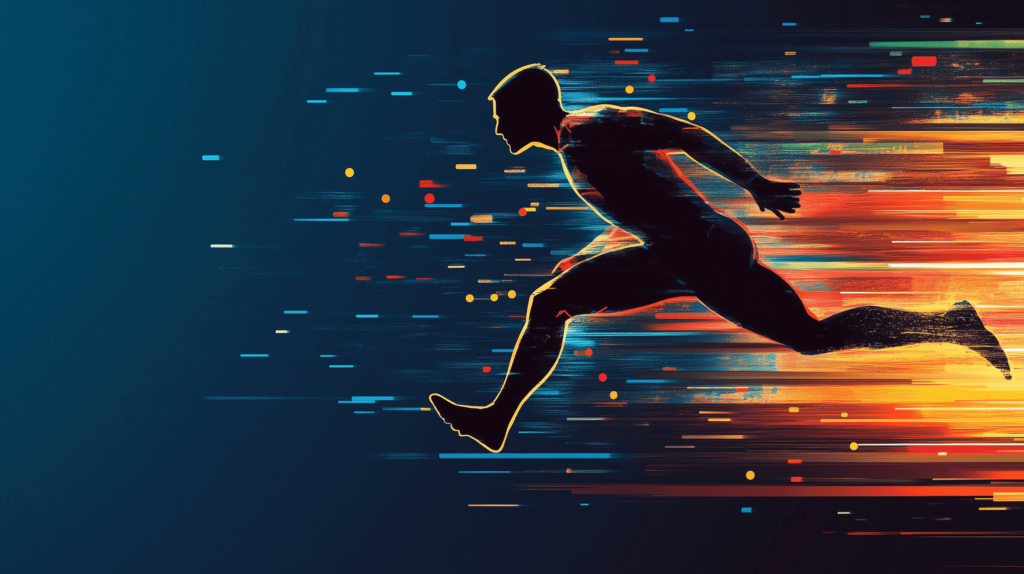
Indexing: The Key to Query Performance
One of the most effective ways to optimize a MySQL database is by using column indexes. MySQL’s default B-tree index excels at exact matches and range searches, improving performance for both WHERE clauses and joins. Proper indexing reduces I/O operations and minimizes overhead, but it’s important to avoid common pitfalls. For example:
- Using functions (e.g., SUBSTRING()) or calculations on indexed columns prevents the index from being utilized, as the function must process every row instead of relying on the index.
To address this, it’s often worth trading off space for time by storing precomputed values in an auxiliary column and indexing that column. For instance, if SUBSTRING(a_column, 1, 4) is frequently used in WHERE clauses or joins, consider creating a separate column to store the precomputed substring values. Index this column, and then use it for filtering and joining instead of recalculating the substring for each query.
Although this approach uses additional storage space, it can significantly improve query performance by reducing computational overhead and allowing MySQL to fully leverage its indexing capabilities. This tradeoff is particularly beneficial when optimizing frequently run queries or those executed on large datasets.
Connection Pooling: Managing Database Connections
Efficient connection management is just as critical as query optimization. Connection pooling maintains a pool of reusable connections, minimizing the overhead of creating new ones for each query. Best practices include:
- Setting a pool size large enough for normal traffic.
- Allowing temporary overflow connections for traffic spikes.
- Recycling idle connections to prevent issues with stale connections.
- Validating connections before use to avoid errors from broken connections.
If you are using SQLAlchemy, you can specify
SQLALCHEMY_ENGINE_OPTIONS = {
‘pool_size’: xxx, # Number of connections in the pool
‘max_overflow’: yyy, # Maximum number of overflow connections
‘pool_recycle’: zzz, # Recycle connections every zzz seconds
‘pool_timeout’: abc, # Timeout after abc seconds if no connection is available
‘pool_pre_ping’:True # Ensure connections are valid before using them (test connections for liveness upon each checkout)
}
Sharepon’s Approach
At Sharepon, these optimization techniques significantly enhanced database performance, reliability, and scalability. By combining well-designed indexes and efficient connection pooling, Sharepon delivers a responsive experience, even during fluctuating loads.
I hope you found this article helpful! Whether you’re building a new web app or optimizing an existing one, MySQL’s versatility and performance make it a reliable foundation for success.