When building a web application, we often focus on the core features that bring users in, such as posting content, sharing links, liking posts, and searching. But as your app starts to grow and real users begin to interact with it, one thing becomes very clear: security is not optional. It’s not just a layer you bolt on after things break. It’s a foundation you lay early, to protect both your users and your platform.
In this post, I’ll walk through how Sharepon, the community-based coupon-sharing app I built, handles user input securely. These insights come from real lessons learned and from small bugs to security design decisions, which will be especially useful to developers building web apps in Python Flask or any framework where users can submit content.
Why Input Security Matters for a Community App
Sharepon is built on the belief that users want to help each other. People post discounts not for profit, but to save others from paying full price. Still, even in a kind and well-intentioned community, security concerns are real. One careless mistake or one malicious actor can jeopardize the experience for everyone.
Unchecked user input can lead to broken pages, impersonation, or far worse — stolen sessions and exposed data. That’s why security matters so much in community-driven apps. Trust is everything. If users can’t rely on the platform to keep things safe, they’ll stop contributing. And when contributions stop, the community falls apart.
So even though I want to believe the best of my users, I design for the worst. And that’s a mindset I would recommend every web developer to adopt.
Preventing XSS with Escaping and Sanitization
One of the most common threats in web apps is Cross-Site Scripting (XSS). This is when an attacker inserts JavaScript into a page, hoping it will run in another user’s browser. If successful, the attacker can hijack sessions, redirect users to fake login pages, or impersonate them outright.
Fortunately, Python Flask uses Jinja2 as its templating engine, which escapes HTML characters by default. That means when someone submits <script>, it becomes harmless text like <script> in the browser. This auto-escaping feature neutralizes a big class of XSS attacks.
But there are times when you need to override it, and that’s where caution is critical.
Early on, Sharepon users asked if they could directly click on links shared by others instead of copying and pasting the URLs manually. To do that, I had to tell Jinja2 not to escape certain content, allowing it to render as raw HTML. That gave users the feature they wanted, but it also put the responsibility for escaping back on me.
To make this safe, I used the escape() function from the markupsafe module to sanitize everything except the links. Then, I wrote custom logic to detect and wrap URLs in <a href> tags. This way, only verified link content gets rendered as HTML, and the rest remains escaped.
This was a good reminder that even small convenience features can introduce new attack surfaces if not handled carefully.
Defending Against CSRF with Flask-WTF
Another common vulnerability is Cross-Site Request Forgery (CSRF). This occurs when a user is tricked into performing an unwanted action on a site where they’re logged in, such as deleting their account or submitting a form.
Sharepon uses Flask-WTF for all form handling, which is the most common way for Flask apps to pass data from front end to backend. And thankfully, this method provides built-in CSRF protection. Once you define a secret key in the app configuration, you can code to include a hidden CSRF token in every form. When a user submits a form, you can validate the form using form.validate_on_submit() to check that the token is present and is valid.
Sharepon enables CSRF protection for every form by default. Even if an attacker tries to mimic a POST request, their request will be missing or have an invalid CSRF token, and Flask will reject it. The best part is, enabling it is just a few lines of code. The payoff, in terms of peace of mind, is enormous.
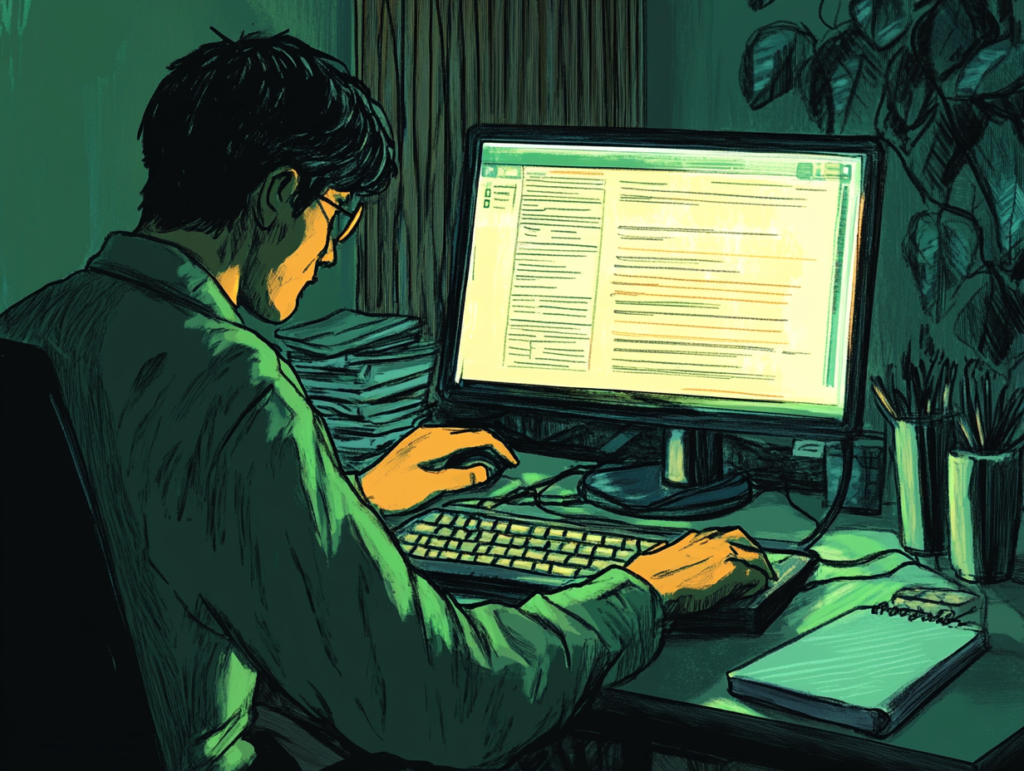
Validating User Input to Protect Structure and Experience
At first glance, input validation might seem like a usability concern — making sure a user types a real email address or that their comment isn’t too long. But in reality, validation is one of the most effective ways to block malformed data, limit abuse, and guide users toward safe, expected behavior.
When Sharepon first launched, I assumed most people would use the platform casually and follow the rules. But users are always more creative than you expect, especially when it comes to edge cases.
A good example is what happened with usernames. I allowed users to pick any name they liked and didn’t restrict on the type of characters. One of my friends created a username that included a forward slash. That slash ended up breaking their profile page URL because the app used the username as part of the URL. What should have been /user/john-smith if he uses a ‘-’ became /user/john/smith as he picked ‘/’ instead, which Flask interpreted as a completely different path. As a result, his homepage became unreachable.
After that incident, I wrote a custom validator to restrict usernames to only alphanumeric characters, underscores, and hyphens.
I also implemented field length limits across the app from the very beginning, not only to protect the database from excessive input, but also to reduce the risk of injection attacks.
Beyond usernames, every form field in Sharepon uses proper validation. Email inputs are checked for format, while text areas have maximum lengths. These small checks add up to a much more secure and stable application.
Final Thoughts
Security isn’t about assuming that users will try to break things. It’s about recognizing that when you open the door to input, like text, links, usernames, emails, you’re also opening the door to potential risks. The more open and user-driven your app is, the more careful you need to be.
For Sharepon, securing input isn’t just a background task. It’s part of building a trustworthy community. From escaping HTML to validating form data and protecting users against forgery attacks, every layer of defense contributes to the platform’s long-term health.
If you’re building your own Flask app or any app that involves user input, I hope these examples help you take a more thoughtful, proactive approach to security. Remember that features bring users in, but trust keeps them there.